Kiption
ComNet Novice

[VE-ARMY] Gunnery Sergeant [VE-VEEC] Executive Officer
Post Number: 69
Total Posts: 152
Joined: Jan 2002
Status: Offline
|

Better DHTML Example
|
|
July 7, 2005
12:28:44 AM
|
|
Works in Firefox and partially in IE. Example
1 <html>
2
3 <head>
4
5 <title>Number Puzzle</title>
6
7 <style>
8 td
9 {
10 text-align: center;
11 padding: 0px;
12 border-spacing: 0px;
13 }
14
15 table
16 {
17 padding: 0px;
18 border-spacing: 0px;
19 }
20
21 td.topLeft {
22 border-top: 4px ridge;
23 border-left: 4px ridge;
24 border-right: 1px black solid;
25 border-bottom: 1px black solid;
26 }
27
28 td.top
29 {
30 border-top: 4px ridge;
31 border-left: 1px black solid;
32 border-right: 1px black solid;
33 border-bottom: 1px black solid;
34 }
35
36 td.topRight
37 {
38 border-top: 4px ridge;
39 border-left: 1px black solid;
40 border-right: 4px ridge;
41 border-bottom: 1px black solid;
42 }
43
44 td.left
45 {
46 border-top: 1px black solid;
47 border-left: 4px ridge;
48 border-right: 1px black solid;
49 border-bottom: 1px black solid;
50 }
51
52 td.middle
53 {
54 border-top: 1px black solid;
55 border-left: 1px black solid;
56 border-right: 1px black solid;
57 border-bottom: 1px black solid;
58 }
59
60 td.right
61 {
62 border-top: 1px black solid;
63 border-left: 1px black solid;
64 border-right: 4px ridge;
65 border-bottom: 1px black solid;
66 }
67
68 td.botLeft
69 {
70 border-top: 1px black solid;
71 border-left: 4px ridge;
72 border-right: 1px black solid;
73 border-bottom: 4px ridge;
74 }
75
76 td.bot
77 {
78 border-top: 1px black solid;
79 border-left: 1px black solid;
80 border-right: 1px black solid;
81 border-bottom: 4px ridge;
82 }
83
84 td.botRight
85 {
86 border-top: 1px black solid;
87 border-left: 1px black solid;
88 border-right: 4px ridge;
89 border-bottom: 4px ridge;
90 }
91
92 td:hover
93 {
94 background-color: red;
95 color: white;
96 }
97
98 </style>
99
100 </head>
101
102
103 <body>
104
105 <table rules="all">
106 <tr>
107 <td id="00" class="topLeft" width="25" onClick="processMove(this.id);"> </td>
108 <td id="01" class="top" width="25" onClick="processMove(this.id);"> </td>
109 <td id="02" class="top" width="25" onClick="processMove(this.id);"> </td>
110 <td id="03" class="topRight" width="25" onClick="processMove(this.id);"> </td>
111 </tr>
112 <tr>
113 <td id="10" class="left" width="25" onClick="processMove(this.id);"> </td>
114 <td id="11" class="middle" width="25" onClick="processMove(this.id);"> </td>
115 <td id="12" class="middle" width="25" onClick="processMove(this.id);"> </td>
116 <td id="13" class="right" width="25" onClick="processMove(this.id);"> </td>
117 </tr>
118 <tr>
119 <td id="20" class="left" width="25" onClick="processMove(this.id);"> </td>
120 <td id="21" class="middle" width="25" onClick="processMove(this.id);"> </td>
121 <td id="22" class="middle" width="25" onClick="processMove(this.id);"> </td>
122 <td id="23" class="right" width="25" onClick="processMove(this.id);"> </td>
123 </tr>
124 <tr>
125 <td id="30" class="botLeft" width="25" onClick="processMove(this.id);"> </td>
126 <td id="31" class="bot" width="25" onClick="processMove(this.id);"> </td>
127 <td id="32" class="bot" width="25" onClick="processMove(this.id);"> </td>
128 <td id="33" class="botRight" width="25" onClick="processMove(this.id);"> </td>
129 </tr>
130 </table>
131
132 <script language="JavaScript">
133
134 var gameBoard = new Array(
135 new Array( 1, 2, 3, 4),
136 new Array( 5, 6, 7, 8),
137 new Array( 9, 10, 11, 12),
138 new Array(13, 14, 15, 0)
139 );
140
141 var zeroLocation = new Array (3, 3);
142
143 function shuffleBoard ()
144 {
145 var numberOfMoves = Math.floor(Math.random() * 256)+256;
146 var zeroLocation = parent.zeroLocation;
147 var gameBoard = parent.gameBoard;
148
149 //Way to actually move pieces around the board is to find the
150 //location of the zero, pick a valid direction, and then swap
151 //the pieces. Fast way of doing this is always remembering
152 //where the zero is.
153
154 for (i = 0; i < numberOfMoves; i++)
155 {
156 var direction = Math.floor(Math.random() * 4);
157
158
159 if (direction == 0 && zeroLocation[0] != 0)
160 {
161 // Up
162 var newValue = gameBoard[zeroLocation[0]-1][zeroLocation[1]];
163 gameBoard[zeroLocation[0]][zeroLocation[1]] = newValue;
164 zeroLocation[0]--;
165 gameBoard[zeroLocation[0]][zeroLocation[1]] = 0;
166 }
167 else if (direction == 1 && zeroLocation[0] != 3)
168 {
169 // Down
170 var newValue = gameBoard[zeroLocation[0]+1][zeroLocation[1]];
171 gameBoard[zeroLocation[0]][zeroLocation[1]] = newValue;
172 zeroLocation[0]++;
173 gameBoard[zeroLocation[0]][zeroLocation[1]] = 0;
174 }
175 else if (direction == 2 && zeroLocation[1] != 0)
176 {
177 // Left
178 var newValue = gameBoard[zeroLocation[0]][zeroLocation[1]-1];
179 gameBoard[zeroLocation[0]][zeroLocation[1]] = newValue;
180 zeroLocation[1]--;
181 gameBoard[zeroLocation[0]][zeroLocation[1]] = 0;
182 }
183 else if (direction == 3 && zeroLocation[1] != 3)
184 {
185 // Right
186 var newValue = gameBoard[zeroLocation[0]][zeroLocation[1]+1];
187 gameBoard[zeroLocation[0]][zeroLocation[1]] = newValue;
188 zeroLocation[1]++;
189 gameBoard[zeroLocation[0]][zeroLocation[1]] = 0;
190 }
191 else
192 {
193 i--;
194 }
195 }
196
197 parent.gameBoard = gameBoard;
198 parent.zeroLocation = zeroLocation;
199
200 }
201
202 function canSwap (xLocation, yLocation)
203 {
204 var zeroLocation = parent.zeroLocation;
205
206 return (zeroLocation[1] == yLocation
207 && (Math.abs(zeroLocation[0] - xLocation) == 1) ||
208 zeroLocation[0] == xLocation
209 && (Math.abs(zeroLocation[1] - yLocation) == 1));
210 }
211
212 function processMove (tdID)
213 {
214 var zeroLocation = parent.zeroLocation;
215 var gameBoard = parent.gameBoard;
216
217 // Need to get the location that was clicked on
218 var xLocation = parseInt(tdID.charAt(0));
219 var yLocation = parseInt(tdID.charAt(1));
220
221 if (canSwap(xLocation, yLocation))
222 {
223 var value = gameBoard[xLocation][yLocation];
224 gameBoard[xLocation][yLocation] = 0;
225 gameBoard[zeroLocation[0]][zeroLocation[1]] = value;
226 zeroLocation[0] = xLocation;
227 zeroLocation[1] = yLocation;
228 writeBoard();
229
230 if (winner())
231 {
232 alert("Yay!! you accomplished something!!");
233 }
234 }
235
236 parent.gameBoard = gameBoard;
237 parent.zeroLocation = zeroLocation;
238 }
239
240 function winner ()
241 {
242 var gameBoard = parent.gameBoard;
243 return gameBoard[0][0] == 1 &&
244 gameBoard[0][1] == 2 &&
245 gameBoard[0][2] == 3 &&
246 gameBoard[0][3] == 4 &&
247 gameBoard[1][0] == 5 &&
248 gameBoard[1][1] == 6 &&
249 gameBoard[1][2] == 7 &&
250 gameBoard[1][3] == 8 &&
251 gameBoard[2][0] == 9 &&
252 gameBoard[2][1] == 10 &&
253 gameBoard[2][2] == 11 &&
254 gameBoard[2][3] == 12 &&
255 gameBoard[3][0] == 13 &&
256 gameBoard[3][1] == 14 &&
257 gameBoard[3][2] == 15 &&
258 gameBoard[3][3] == 0;
259 }
260
261 function writeBoard ()
262 {
263 gameBoard = parent.gameBoard;
264 for (i = 0; i < gameBoard.length; i++)
265 {
266 for (j = 0; j < gameBoard[i].length; j++)
267 {
268 var elementID = i+""+j;
269 var element = document.getElementById(elementID);
270 var value = gameBoard[i][j];
271 if (value == 0)
272 {
273 value = "";
274 }
275
276 element.replaceChild(
277 document.createTextNode(value),
278 element.firstChild);
279 }
280 }
281 }
282
283 shuffleBoard();
284 writeBoard();
285
286 </script>
287 </body>
288 </html>
289
Again, I'm not going to waste time explaining it, unless asked.
-----------------------
Just another pretty face....
[This message has been edited by
Kiption
(edited July 7, 2005
12:31:00 AM)]
|
Yillis
ComNet Cadet
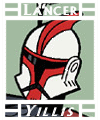
[VE-ARMY] Lance Corporal [VE-VEEC] Gaming Reporter
Post Number: 263
Total Posts: 1598
Joined: Mar 2005
Status: Offline
|

RE: Better DHTML Example
|
|
July 7, 2005
4:00:43 AM
|
|
But what do you do? Like whats the point of the puzzle? ----------------------- Lance Corporal Yillis
-=Lancer Squad=- -=ASL=- -=Moonbeam=-
ASL/LCPL Yillis/2SQD/2PLT/1COM/1RGT/1BAT/VEA /VE /Tadath [LoR] [WM]
|
Hunter Emnis
ComNet Initiate

[VE-ARMY] Private First Class
Post Number: 171
Total Posts: 407
Joined: May 2005
Status: Offline
|

RE: Better DHTML Example
|
|
July 7, 2005
7:07:18 AM
|
|
srry
-----------------------
HUnter EMnis
"Combine self-hate with pride,
a leader with a follower,
seriousness with immaturity,
smartness with idiocy,
merciful with relentlessness,
a friend with an enemy.
Combine two opposites,
and in the middle,
[This message has been edited by
Hunter Emnis
(edited July 7, 2005
11:59:48 AM)]
|
Angel
ComNet Expert
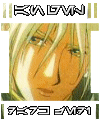
[VE-ARMY] Senior Sergeant [VE-DJO] Dark Jedi Knight
Post Number: 1882
Total Posts: 3342
Joined: Jul 2003
Status: Offline
|

RE: Better DHTML Example
|
|
July 7, 2005
11:56:18 AM
|
|
The point is to put the numbers in order.
Hunter: shut up. Kip does his own programming. You are addressing a former Head of the High Council. You would do well to bite your tongue. ----------------------- Senior Sergeant Jikkyo "Angel" Nimiichi
=[Arrogant Elitist]=
AS-2/ASL/SSG Jikkyo "Angel" Nimiichi/Iron Horse Squad/WildCard/Phoenix/Dragon/ Osiris/Army/VE [LoR] [RoM] [BC] [CDS] [WM] [GRoM] {BoA}
------------------------
The Angel of Death
CM/DJK Jikkyo "Angel" Nimiichi/Eagle 1-1/Sith/VEDJ/VE/Lopen [VP:1]
------------------------
He who sheds man's blood, by man shall his blood be shed. The three shall spread their blackened wings and be the vengeful striking hammer of God..
|
Kairo
ComNet Member
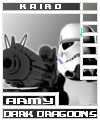
[VE-ARMY] Senior Sergeant
Post Number: 568
Total Posts: 1338
Joined: Jun 2003
Status: Offline
|

RE: Better DHTML Example
|
|
July 7, 2005
1:52:26 PM
|
|
Are you telling me you've never done one of those before Yillis? Oh, and for this one, if anyone cares to know, I did it in thirty seconds, give or take a second...
-----------------------
Senior Sergeant Kairo
Squad Leader
*DarkDragoons*
SL/SR.SGT Kairo/2SQD/1PLT/1COMP/1BAT/1RGT/VEA/VE [CDS][BoA][CoR]
Know your enemy and know yourself, and you will be victorious in ten thousand battles.
You may forget the past, but it always has a way of haunting your future.
Men can die, but a symbol is eternal.
[This message has been edited by
Kairo
(edited July 8, 2005
7:52:11 AM)]
|
Japheth
ComNet Expert
[VE-DJO] Krath Templar [VE-NAVY] Admiral [VE-VEHC] Admiral*
Post Number: 1613
Total Posts: 2517
Joined: Nov 2001
Status: Offline
|

RE: Better DHTML Example
|
|
July 7, 2005
2:45:03 PM
|
|
It actually "randomly" generates the board each time you reload. ----------------------- Admiral Japheth Cappadocious, Krath Templar
Naval Commander in Chief, Captain of the mSSD Atrus
Headmaster of the Dark Jedi Order Academy
-----------------------
NCC/ADM Japheth Cappadocious/NHC-1/Raptor 1/mSSD Atrus/DEF/VEN/VE/(=MA=)(=SCPA=)(=FCO=)[BRC][LSM][MC:1][KC:OC][IGC][MoH]
HM/KT Japheth Cappadocious/DC-3/Krath Order/Elite Griffen Sect/VEDJ/VE/[SoY][EoP]
|
Kairo
ComNet Member
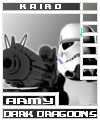
[VE-ARMY] Senior Sergeant
Post Number: 570
Total Posts: 1338
Joined: Jun 2003
Status: Offline
|

RE: Better DHTML Example
|
|
July 7, 2005
6:05:42 PM
|
|
I understand that, and I'm normally really good with those types of puzzles. ----------------------- Senior Sergeant Kairo
Squad Leader
*DarkDragoons*
SL/SR.SGT Kairo/2SQD/1PLT/1COMP/1BAT/1RGT/VEA/VE [CDS][BoA][CoR]
Know your enemy and know yourself, and you will be victorious in ten thousand battles.
You may forget the past, but it always has a way of haunting your future.
Men can die, but a symbol is eternal.
|
Kiption
ComNet Novice

[VE-ARMY] Gunnery Sergeant [VE-VEEC] Executive Officer
Post Number: 73
Total Posts: 152
Joined: Jan 2002
Status: Offline
|

RE: Better DHTML Example
|
|
July 7, 2005
9:07:37 PM
|
|
Awww, I wanna see what he has to say....
----------------------- Just another pretty face....
|
Merrick
ComNet Member
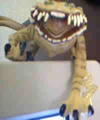
[VE-ARMY] 1st Lieutenant
Post Number: 371
Total Posts: 659
Joined: Feb 2002
Status: Offline
|

RE: Better DHTML Example
|
|
July 7, 2005
9:11:38 PM
|
|
Such a wimp Hunter. At least have the conviction to leave your stupid responses there if you are going to post them in the first place. ----------------------- Jester Squad
XO/1LT Merrick/VEA/TADATH/VE [CotE][SoA][CoH]
Army Executive Officer
Verastinian Republic - Minister of Subversion
-----------------------
To thy protection fear and sorrow flee, and those that weary are of light find rest in thee.
If you love something, set it free. If it doesn't come back, hunt it down and kill it.
|
Hunter Emnis
ComNet Cadet

[VE-ARMY] Private First Class
Post Number: 203
Total Posts: 407
Joined: May 2005
Status: Offline
|

RE: Better DHTML Example
|
|
July 8, 2005
6:47:57 AM
|
|
I am not afraid for people to read it, but I find it annoying about people bugging me about what i said. And kiption do you have anymore? Enlighten us. ----------------------- HUnter EMnis
"Combine self-hate with pride,
a leader with a follower,
seriousness with immaturity,
smartness with idiocy,
merciful with relentlessness,
a friend with an enemy.
Combine two opposites,
and in the middle,
|
Dez
ComNet Initiate
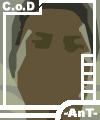
[VE-ARMY] 1st Lieutenant
Post Number: 154
Total Posts: 371
Joined: May 2001
Status: Offline
|

RE: Better DHTML Example
|
|
July 12, 2005
10:09:29 AM
|
|
"far end object equals to check..... nananana"
there's tons of typo there..
bleh.
geek
|